Hey guys.!
Here i'm going to show how to do crud operation using grid.
In this article I have used Poco and resource files.
So, lets Start.
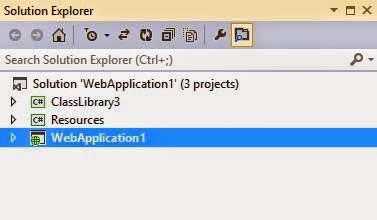
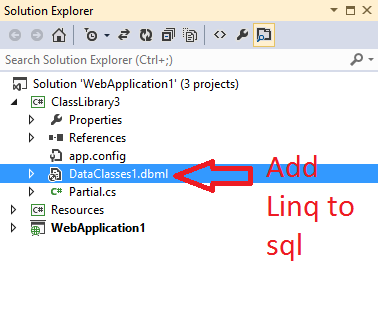
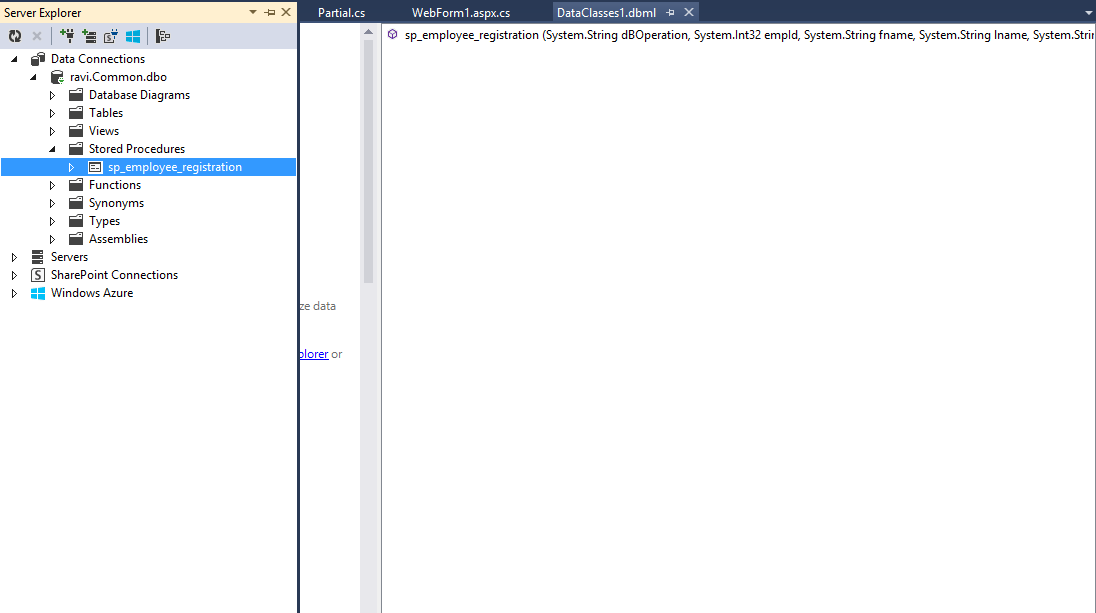
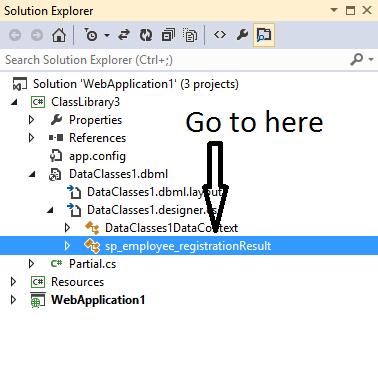
And copy Partial class.
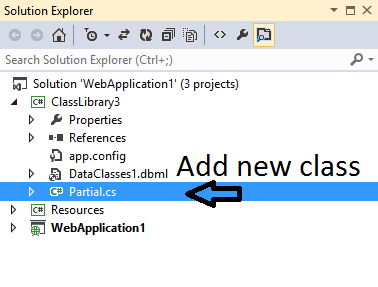
Now Replace class Partial with the partial class code in Partial .cs .
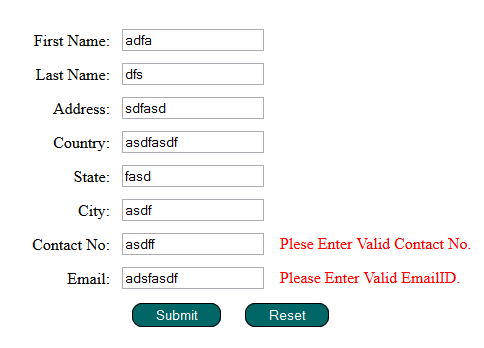
Thank you .Feel free for comment.
Dowload Source From Here
Here i'm going to show how to do crud operation using grid.
In this article I have used Poco and resource files.
So, lets Start.
Step:1
Here create one table named employee_registration Script is given below.
USE [Common]
GO
/******
Object: Table
[dbo].[employee_registration] Script
Date: 04/14/2014 16:57:41 ******/
SET ANSI_NULLS ON
GO
SET QUOTED_IDENTIFIER ON
GO
CREATE TABLE [dbo].[employee_registration](
[empId] [int] IDENTITY(1,1) NOT NULL,
[fname] [nvarchar](50) NULL,
[lname] [nvarchar](50) NULL,
[address] [nvarchar](50) NULL,
[country] [nvarchar](50) NULL,
[state] [nvarchar](50) NULL,
[city] [nvarchar](50) NULL,
[contactno] [nvarchar](50) NULL,
[email] [nvarchar](50) NULL,
CONSTRAINT
[PK_ravi_employee_registration] PRIMARY KEY CLUSTERED
(
[empId] ASC
)WITH
(PAD_INDEX
= OFF, STATISTICS_NORECOMPUTE = OFF, IGNORE_DUP_KEY = OFF, ALLOW_ROW_LOCKS
= ON, ALLOW_PAGE_LOCKS = ON) ON [PRIMARY]
) ON [PRIMARY]
GO
Step:2
Now Create Stored Procedure fro crud operation purpose.Script given below
USE [Common]
GO
/******
Object: StoredProcedure
[dbo].[sp_employee_registration]
Script Date: 04/14/2014 16:24:21 ******/
SET ANSI_NULLS ON
GO
SET QUOTED_IDENTIFIER ON
GO
--
=============================================
-- Author: Ravi
-- Create date:
12 April 2014
-- Description: sp_employee_registration
--
=============================================
CREATE PROCEDURE [dbo].[sp_employee_registration]
@DBOperation varchar(50),
@empId int,
@fname nvarchar(50),
@lname nvarchar(50),
@address nvarchar(50),
@country nvarchar(50),
@state nvarchar(50),
@city nvarchar(50),
@contactno nvarchar(50),
@email nvarchar(50),
@ErrorMsg nvarchar(MAX)
AS
BEGIN
BEGIN TRY
IF(@DBOperation='Create')
BEGIN
INSERT INTO [Common].[dbo].[employee_registration]
([fname]
,[lname]
,[address]
,[country]
,[state]
,[city]
,[contactno]
,[email])
VALUES
(@fname
,@lname
,@address
,@country
,@state
,@city
,@contactno
,@email);
SELECT *
FROM [Common].[dbo].[employee_registration]
WHERE empId=SCOPE_IDENTITY();
END
ELSE IF(@DBOperation='ReadAll')
BEGIN
SELECT *
FROM [Common].[dbo].[employee_registration];
END
ELSE IF(@DBOperation='ReadSpecific')
BEGIN
SELECT *
FROM [Common].[dbo].[employee_registration]
WHERE empId=@empId;
END
ELSE IF(@DBOperation='Update')
BEGIN
UPDATE
[Common].[dbo].[employee_registration]
SET [fname] = @fname
,[lname] =
@lname
,[address] =
@address
,[country] =
@country
,[state] =
@state
,[city] =
@city
,[contactno] =
@contactno
,[email] =
@email
WHERE empId=@empId;
SELECT*
FROM [Common].[dbo].[employee_registration]
WHERE empId=@empId;
END
ELSE IF(@DBOperation='Delete')
BEGIN
DELETE FROM [Common].[dbo].[employee_registration]
WHERE empId=@empId;
SELECT *
FROM [Common].[dbo].[employee_registration]
WHERE empId=@empId;
END
END TRY
BEGIN CATCH
SET @ErrorMsg
= error_message();
RAISERROR(@ErrorMsg,11,12);
END CATCH
END
Step :3
Now open visual Studio and Create new project .and add Webapplication
also add two new projects(Class Library) in same solution .
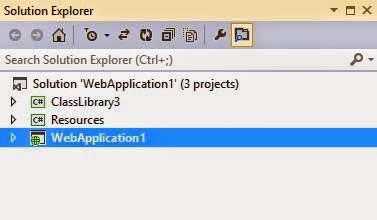
Now add Linq to sql in Class Library
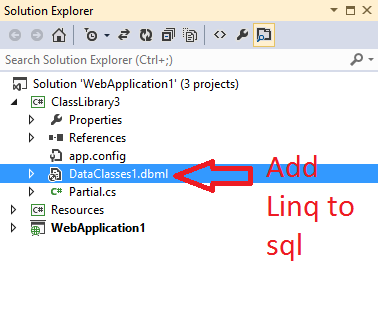
Now drag and drop created StoredProcedure.
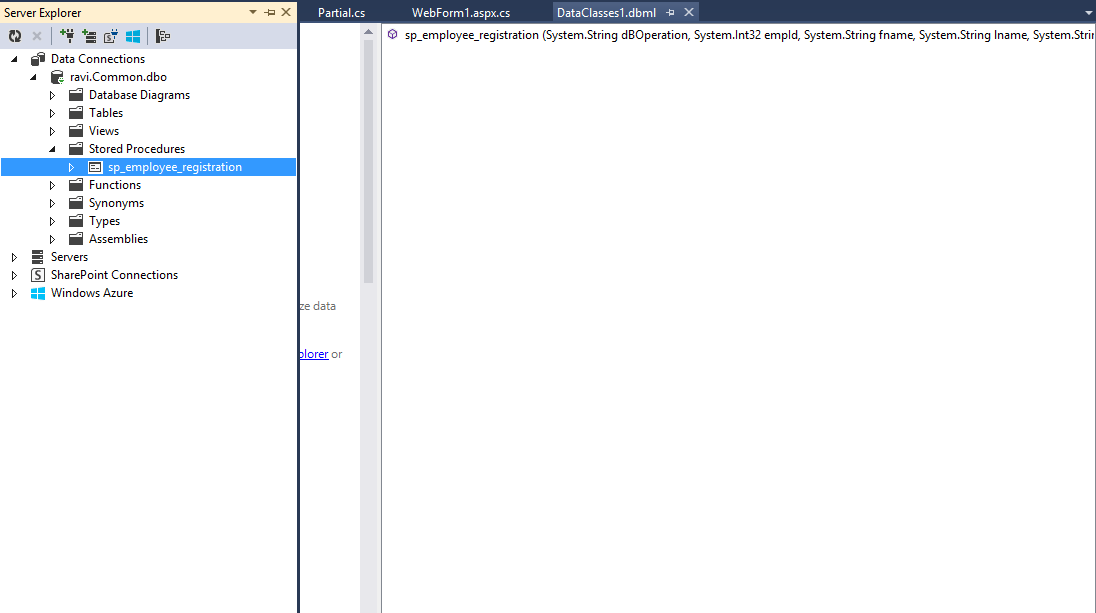
Now Opne sp_employee_registrationResult.
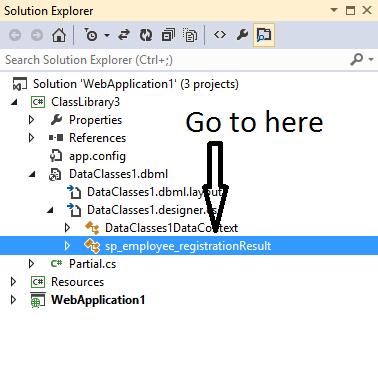
And copy Partial class.
Now add new Class file in ClassLibrary 3 Project and give name Partial
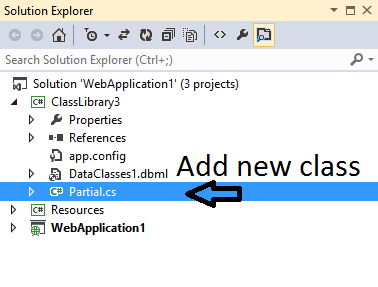
Now Replace class Partial with the partial class code in Partial .cs .
using System;
using System.Collections.Generic;
using System.Data.SqlClient;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Resources;
namespace ClassLibrary3
{
public partial class sp_employee_registrationResult
{
#region
Declaration
public string dbOperationMessage { get; set; }
public string dboperationStatus { get; set; }
#endregion
}
Step:4
Now Go to Resource Project.and add three resource files .
- dbOpeartionMsgResource.resx
- dbOpeartionResource.resx
- dbOperationStatusResource.resx
In dbOpeartionMsgResource.resx:
Now Change Access Modifier from Internal to Public.
Now goto ClassLibrary 3 And add Reference of Resource Project.
Now here into the partial.cs Class we create CRUD Operation.Code gien below
using System;
using System.Collections.Generic;
using System.Data.SqlClient;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Resources;
namespace ClassLibrary3
{
public partial class sp_employee_registrationResult
{
#region
Declaration
public string dbOperationMessage { get; set; }
public string dboperationStatus { get; set; }
#endregion
public List<sp_employee_registrationResult> CreateEmployee(sp_employee_registrationResult empList)
{
List<sp_employee_registrationResult> CreateEmployeeList = new List<sp_employee_registrationResult>();
try
{
using (DataClasses1DataContext db = new DataClasses1DataContext())
{
CreateEmployeeList = db.sp_employee_registration(dbOpeartionResource.Create,
null, empList.fname,
empList.lname, empList.address, empList.country, empList.state, empList.city,
empList.contactno, empList.email, null).ToList();
CreateEmployeeList[0].dboperationStatus = dbOperationStatusResource.Succes;
CreateEmployeeList[0].dbOperationMessage = string.Format("Employee'" +
empList.fname + "'{0}", dbOpeartionMsgResource.Created);
}
}
catch (SqlException SqlEx)
{
CreateEmployeeList.Add(new sp_employee_registrationResult { dboperationStatus = dbOperationStatusResource.Failed, dbOperationMessage = string.Format("Message : {0} <br /> Linenumber:{1} <br
/> Procedurename:{2} ", dbOperationStatusResource.Failed,
SqlEx.LineNumber, SqlEx.Procedure) });
}
catch (Exception Ex)
{
CreateEmployeeList.Add(new sp_employee_registrationResult { dboperationStatus = dbOperationStatusResource.Failed, dbOperationMessage = string.Format("Message : {0} <br /> Exception:{0} ", dbOperationStatusResource.Failed, Ex.Message) });
}
return CreateEmployeeList;
}
public List<sp_employee_registrationResult> ReadAllEmployee()
{
List<sp_employee_registrationResult> ReadAllList = new List<sp_employee_registrationResult>();
try
{
using (DataClasses1DataContext db = new DataClasses1DataContext())
{
ReadAllList =
db.sp_employee_registration(dbOpeartionResource.ReadAll, null, null, null, null, null, null, null, null, null, null).ToList();
ReadAllList[0].dboperationStatus
= dbOperationStatusResource.Succes;
}
}
catch (SqlException SqlEx)
{
ReadAllList.Add(new sp_employee_registrationResult { dboperationStatus = dbOperationStatusResource.Failed, dbOperationMessage = string.Format("Message : {0} <br /> Linenumber:{1} <br
/> Procedurename:{2} ", dbOperationStatusResource.Failed,
SqlEx.LineNumber, SqlEx.Procedure) });
}
catch (Exception Ex)
{
ReadAllList.Add(new sp_employee_registrationResult { dboperationStatus = dbOperationStatusResource.Failed, dbOperationMessage = string.Format("Message : {0} <br /> Exception:{0} ", dbOperationStatusResource.Failed, Ex.Message) });
}
return ReadAllList;
}
public List<sp_employee_registrationResult> ReadSpecificEmployee(int empId)
{
List<sp_employee_registrationResult> ReadSpecificList = new List<sp_employee_registrationResult>();
try
{
using (DataClasses1DataContext db = new DataClasses1DataContext())
{
ReadSpecificList =
db.sp_employee_registration(dbOpeartionResource.ReadSpecific, empId, null, null, null, null, null, null, null, null, null).ToList();
ReadSpecificList[0].dboperationStatus = dbOperationStatusResource.Succes;
}
}
catch (SqlException SqlEx)
{
ReadSpecificList.Add(new sp_employee_registrationResult { dboperationStatus = dbOperationStatusResource.Failed, dbOperationMessage = string.Format("Message : {0} <br /> Linenumber:{1} <br
/> Procedurename:{2} ", dbOperationStatusResource.Failed,
SqlEx.LineNumber, SqlEx.Procedure) });
}
catch (Exception Ex)
{
ReadSpecificList.Add(new sp_employee_registrationResult { dboperationStatus = dbOperationStatusResource.Failed, dbOperationMessage = string.Format("Message : {0} <br /> Exception:{0} ", dbOperationStatusResource.Failed, Ex.Message) });
}
return ReadSpecificList;
}
public List<sp_employee_registrationResult> DeleteEmployee(int empId)
{
List<sp_employee_registrationResult> DeleteList = new List<sp_employee_registrationResult>();
try
{
using (DataClasses1DataContext db = new DataClasses1DataContext())
{
DeleteList =
db.sp_employee_registration(dbOpeartionResource.Delete, empId, null, null, null, null, null, null, null, null, null).ToList();
DeleteList[0].dboperationStatus = dbOperationStatusResource.Succes;
}
}
catch (SqlException SqlEx)
{
DeleteList.Add(new sp_employee_registrationResult { dboperationStatus = dbOperationStatusResource.Failed, dbOperationMessage = string.Format("Message : {0} <br /> Linenumber:{1} <br
/> Procedurename:{2} ", dbOperationStatusResource.Failed,
SqlEx.LineNumber, SqlEx.Procedure) });
}
catch (Exception Ex)
{
DeleteList.Add(new sp_employee_registrationResult { dboperationStatus = dbOperationStatusResource.Failed, dbOperationMessage = string.Format("Message : {0} <br /> Exception:{0} ", dbOperationStatusResource.Failed, Ex.Message) });
}
return DeleteList;
}
public List<sp_employee_registrationResult> UpdateEmployee(sp_employee_registrationResult empList)
{
List<sp_employee_registrationResult> UpdateList = new List<sp_employee_registrationResult>();
try
{
using (DataClasses1DataContext db = new DataClasses1DataContext())
{
UpdateList =
db.sp_employee_registration(dbOpeartionResource.Update, empId, empList.fname, empList.lname,
empList.address, empList.country, empList.state, empList.city,
empList.contactno, empList.email, null).ToList();
UpdateList[0].dboperationStatus = dbOperationStatusResource.Succes;
}
}
catch (SqlException SqlEx)
{
UpdateList.Add(new sp_employee_registrationResult { dboperationStatus = dbOperationStatusResource.Failed, dbOperationMessage = string.Format("Message : {0} <br /> Linenumber:{1} <br
/> Procedurename:{2} ", dbOperationStatusResource.Failed,
SqlEx.LineNumber, SqlEx.Procedure) });
}
catch (Exception Ex)
{
UpdateList.Add(new sp_employee_registrationResult { dboperationStatus = dbOperationStatusResource.Failed, dbOperationMessage = string.Format("Message : {0} <br /> Exception:{0} ", dbOperationStatusResource.Failed, Ex.Message) });
}
return UpdateList;
}
}
}
Now got to webapplication and paste given code into WeFrom1.aspx:
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="WebForm1.aspx.cs" Inherits="WebApplication1.WebForm1" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
<style type="text/css">
.gv
{
font-family: Arial;
margin-top: 30px;
font-size: 14px;
}
.gv th
{
background-color:#006666;
font-weight: bold;
color: #fff;
padding: 2px 10px;
}
.gv td
{
padding: 2px 10px;
}
input[type="submit"]
{
margin: 2px 10px;
padding: 2px 20px;
background-color: #006666;
border-radius: 10px;
border: solid 1px #000;
cursor: pointer;
color: #fff;
}
input[type="submit"]:hover
{
background-color: #00BFFF;
}
</style>
</head>
<body>
<form id="form1" runat="server">
<div><br />
<asp:ScriptManager ID="ScriptManager1" runat="server">
</asp:ScriptManager>
<br />
<table width="100%" colspan="4" cellpadding="5">
<tr>
<td style="text-align: right; width: 40%">First Name:</td>
<td style="text-align: left; width: 60%">
<asp:TextBox ID="txt_fname" runat="server" placeholder="First Name"></asp:TextBox>
<asp:RequiredFieldValidator ID="RequiredFieldValidator1" runat="server" ControlToValidate="txt_fname" ErrorMessage="*" ForeColor="Red" ValidationGroup="val"></asp:RequiredFieldValidator>
</td>
</tr>
<tr>
<td style="text-align: right; width: 40%">Last Name:</td>
<td style="text-align: left; width: 60%">
<asp:TextBox ID="txt_lname" runat="server" placeholder="Last Name"></asp:TextBox>
<asp:RequiredFieldValidator ID="RequiredFieldValidator2" runat="server" ControlToValidate="txt_lname" ErrorMessage="*" ForeColor="Red" ValidationGroup="val"></asp:RequiredFieldValidator>
</td>
</tr>
<tr>
<td style="text-align: right; width: 40%">Address:</td>
<td style="text-align: left; width: 60%">
<asp:TextBox ID="txt_address" runat="server" placeholder="Address"></asp:TextBox>
<asp:RequiredFieldValidator ID="RequiredFieldValidator3" runat="server" ControlToValidate="txt_address" ErrorMessage="*" ForeColor="Red" ValidationGroup="val"></asp:RequiredFieldValidator>
</td>
</tr>
<tr>
<td style="text-align: right; width: 40%">Country:</td>
<td style="text-align: left; width: 60%">
<asp:TextBox ID="txt_country" runat="server" placeholder="Country"></asp:TextBox>
<asp:RequiredFieldValidator ID="RequiredFieldValidator4" runat="server" ControlToValidate="txt_country" ErrorMessage="*" ForeColor="Red" ValidationGroup="val"></asp:RequiredFieldValidator>
</td>
</tr>
<tr>
<td style="text-align: right; width: 40%">State:</td>
<td style="text-align: left; width: 60%">
<asp:TextBox ID="txt_state" runat="server" placeholder="State"></asp:TextBox>
<asp:RequiredFieldValidator ID="RequiredFieldValidator5" runat="server" ControlToValidate="txt_state" ErrorMessage="*" ForeColor="Red" ValidationGroup="val"></asp:RequiredFieldValidator>
</td>
</tr>
<tr>
<td style="text-align: right; width: 40%">City:</td>
<td style="text-align: left; width: 60%">
<asp:TextBox ID="txt_city" runat="server" placeholder="City"></asp:TextBox>
<asp:RequiredFieldValidator ID="RequiredFieldValidator6" runat="server" ControlToValidate="txt_city" ErrorMessage="*" ForeColor="Red" ValidationGroup="val"></asp:RequiredFieldValidator>
</td>
</tr>
<tr>
<td style="text-align: right; width: 40%">Contact No:</td>
<td style="text-align: left; width: 60%">
<asp:TextBox ID="txt_contact" runat="server" placeholder="Contact No"></asp:TextBox>
<asp:RequiredFieldValidator ID="RequiredFieldValidator7" runat="server" ControlToValidate="txt_contact" ErrorMessage="*" ForeColor="Red" ValidationGroup="val"></asp:RequiredFieldValidator>
<asp:RegularExpressionValidator ID="RegularExpressionValidator1" runat="server" ControlToValidate="txt_contact" ErrorMessage="Plese Enter Valid Contact No." ForeColor="Red" ValidationExpression="\d{10}" ValidationGroup="val"></asp:RegularExpressionValidator>
</td>
</tr>
<tr>
<td style="text-align: right; width: 40%">Email:</td>
<td style="text-align: left; width: 60%">
<asp:TextBox ID="txt_email" runat="server" placeholder="Email"></asp:TextBox>
<asp:RequiredFieldValidator ID="RequiredFieldValidator8" runat="server" ControlToValidate="txt_email" ErrorMessage="*" ForeColor="Red" ValidationGroup="val"></asp:RequiredFieldValidator>
<asp:RegularExpressionValidator ID="RegularExpressionValidator2" runat="server" ControlToValidate="txt_email" ErrorMessage="Please Enter Valid EmailID." ForeColor="Red" ValidationExpression="\w+([-+.']\w+)*@\w+([-.]\w+)*\.\w+([-.]\w+)*" ValidationGroup="val"></asp:RegularExpressionValidator>
</td>
</tr>
<tr>
<td style="text-align: right; width: 40%">
<asp:Timer ID="Timer1" runat="server" Interval="20000" OnTick="Timer1_Tick">
</asp:Timer>
</td>
<td style="text-align: left; width: 60%">
<asp:Button ID="btn_submit" Text="Submit" runat="server" OnClick="btn_submit_Click" ValidationGroup="val" />
<asp:Button ID="btn_reset" Text="Reset" runat="server" OnClick="btn_reset_Click" />
</td>
</tr>
<tr>
<td style="text-align: right; width: 40%"> </td>
<td style="text-align: left; width: 60%">
<asp:Label ID="lbl_Msg" runat="server"></asp:Label>
</td>
</tr>
</table>
<div align="center">
<asp:GridView ID="GridView" runat="server" AutoGenerateColumns="False" GridLines="both" CssClass="gv" EmptyDataRowStyle-ForeColor="Red" >
<Columns>
<asp:TemplateField HeaderText="First Name">
<ItemTemplate>
<asp:Label ID="Label1" runat="server" Text='<%# Eval("fname") %>' ></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Last Name">
<ItemTemplate>
<asp:Label ID="Label2" runat="server" Text='<%# Eval("lname") %>'></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="address">
<ItemTemplate>
<asp:Label ID="Label3" runat="server" Text='<%# Eval("address") %>'></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="country">
<ItemTemplate>
<asp:Label ID="Label4" runat="server" Text='<%# Eval("country") %>'></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="state">
<ItemTemplate>
<asp:Label ID="Label5" runat="server" Text='<%# Eval("state") %>'></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="city">
<ItemTemplate>
<asp:Label ID="Label6" runat="server" Text='<%# Eval("city") %>'></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="contactno">
<ItemTemplate>
<asp:Label ID="Label7" runat="server" Text='<%# Eval("contactno") %>'></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="email">
<ItemTemplate>
<asp:Label ID="Label8" runat="server" Text='<%# Eval("email") %>'></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Action">
<ItemTemplate>
<asp:LinkButton ID="lkb_edit" runat="server" OnClick="lkb_edit_Click" CommandArgument='<%#Eval("empId") %>'>Edit</asp:LinkButton>
<asp:LinkButton ID="lkb_delete" runat="server" Text="Delete" CommandArgument='<%#Eval("empId") %>' OnClientClick="return
confirm('Are you sure? want to delete the Employee.');" OnClick="lkb_delete_Click" />
</ItemTemplate>
</asp:TemplateField>
</Columns>
<EmptyDataRowStyle ForeColor="Red"></EmptyDataRowStyle>
<FooterStyle BackColor="White" ForeColor="#000066" />
<HeaderStyle BackColor="#006699" Font-Bold="True" ForeColor="White" />
<PagerStyle BackColor="White" ForeColor="#000066" HorizontalAlign="Left" />
<RowStyle ForeColor="#000066" />
<SelectedRowStyle BackColor="#669999" Font-Bold="True" ForeColor="White" />
<SortedAscendingCellStyle BackColor="#F1F1F1" />
<SortedAscendingHeaderStyle BackColor="#007DBB" />
<SortedDescendingCellStyle BackColor="#CAC9C9" />
<SortedDescendingHeaderStyle BackColor="#00547E" />
</asp:GridView>
</div>
</div>
</form>
</body>
</html>
paste below code in WebForm1.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using ClassLibrary3;
using Resources;
namespace WebApplication1
{
public partial class WebForm1 : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
Bindgrid();
}
}
public void Bindgrid()
{
var query= new sp_employee_registrationResult().ReadAllEmployee().ToList();
if (query.Any())
{
if (query[0].dboperationStatus == dbOperationStatusResource.Failed)
{
GridView.DataSource = string.Empty;
GridView.DataBind();
}
else
{
GridView.DataSource =
query;
GridView.DataBind();
}
}
}
protected void btn_submit_Click(object sender, EventArgs e)
{
try
{
if (btn_submit.Text == "Submit")
{
var query = new sp_employee_registrationResult().CreateEmployee(GetControlValues());
if (query[0].dboperationStatus
== dbOperationStatusResource.Failed)
{
lbl_Msg.Text =
query[0].dbOperationMessage;
lbl_Msg.ForeColor =
System.Drawing.Color.Red;
}
else
{
lbl_Msg.Text =
query[0].dbOperationMessage;
lbl_Msg.ForeColor =
System.Drawing.Color.Blue;
}
}
else if (btn_submit.Text == "Update")
{
var query = new sp_employee_registrationResult().UpdateEmployee(GetControlValues());
if (query[0].dboperationStatus
== dbOperationStatusResource.Failed)
{
lbl_Msg.Text =
query[0].dbOperationMessage;
lbl_Msg.ForeColor =
System.Drawing.Color.Red;
}
else
{
lbl_Msg.Text =
query[0].dbOperationMessage;
lbl_Msg.ForeColor = System.Drawing.Color.Blue;
}
}
}
catch (Exception Ex)
{
lbl_Msg.Text = string.Format("Creation Failed ",
Ex.Message);
lbl_Msg.ForeColor =
System.Drawing.Color.Red;
}
finally
{
btn_submit.Text = "Submit";
Bindgrid();
Reset();
Timer1.Enabled = true;
Timer1.Interval = 2000;
}
}
private sp_employee_registrationResult GetControlValues()
{
sp_employee_registrationResult obj = new sp_employee_registrationResult()
{
//empId=
fname = !string.IsNullOrEmpty(txt_fname.Text)
? txt_fname.Text : null,
lname = !string.IsNullOrEmpty(txt_lname.Text)
? txt_lname.Text : null,
address = !string.IsNullOrEmpty(txt_address.Text)
? txt_address.Text : null,
country = !string.IsNullOrEmpty(txt_country.Text)
? txt_country.Text : null,
state = !string.IsNullOrEmpty(txt_state.Text)
? txt_state.Text : null,
city = !string.IsNullOrEmpty(txt_city.Text)
? txt_city.Text : null,
contactno = !string.IsNullOrEmpty(txt_contact.Text)
? txt_contact.Text : null,
email = !string.IsNullOrEmpty(txt_email.Text)
? txt_email.Text : null
};
return obj;
}
protected void btn_reset_Click(object sender, EventArgs e)
{
Reset();
}
private void Reset()
{
btn_submit.Text = "Submit";
txt_fname.Text = string.Empty;
txt_lname.Text = string.Empty;
txt_address.Text = string.Empty;
txt_city.Text = string.Empty;
txt_contact.Text = string.Empty;
txt_country.Text = string.Empty;
txt_state.Text = string.Empty;
txt_email.Text = string.Empty;
}
protected void Timer1_Tick(object sender, EventArgs e)
{
lbl_Msg.Text = string.Empty;
Response.Redirect("WebForm1.aspx");
}
protected void lkb_edit_Click(object sender, EventArgs e)
{
LinkButton lk = (LinkButton)sender;
int empId = Convert.ToInt32(lk.CommandArgument);
ViewState["ID"] =
empId;
SetControl(empId);
}
private void SetControl(int empId)
{
List<sp_employee_registrationResult> obj = new sp_employee_registrationResult().ReadSpecificEmployee(empId).ToList();
txt_fname.Text =
obj.ToList()[0].fname;
txt_lname.Text = obj.ToList()[0].lname;
txt_address.Text =
obj.ToList()[0].address;
txt_city.Text =
obj.ToList()[0].city;
txt_contact.Text =
obj.ToList()[0].contactno;
txt_country.Text =
obj.ToList()[0].country;
txt_state.Text =
obj.ToList()[0].state;
txt_email.Text =
obj.ToList()[0].email;
btn_submit.Text = "Update";
}
protected void lkb_delete_Click(object sender, EventArgs e)
{
LinkButton lk = (LinkButton)sender;
int empId = Convert.ToInt32(lk.CommandArgument);
DeleteEmp(empId);
}
private void DeleteEmp(int empId)
{
var query = new sp_employee_registrationResult().DeleteEmployee(empId);
Bindgrid();
Reset();
}
}
}
Now you have done coding part.for validation purpose place Required field validator in all TextBox Controls.
Now Run the Application(SnapShots):
Click For Delete:
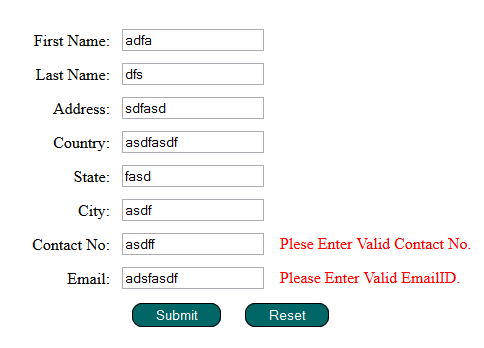
Thank you .Feel free for comment.
Dowload Source From Here